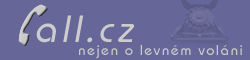 |
/* $Id: config.inc.php3,v 1.17 2000/04/24 14:36:22 tobias Exp $ */
// MySQL hostname
$phpAds_hostname = "localhost";
// MySQL username
$phpAds_mysqluser = "nobody";
// MySQL password
$phpAds_mysqlpassword = "bukvice";
// The database phpAds lives in
$phpAds_db = "phpads";
// phpAds' database tables
$phpAds_tbl_adclicks = "adclicks";
$phpAds_tbl_adviews = "adviews";
$phpAds_tbl_banners = "banners";
$phpAds_tbl_clients = "clients";
$phpAds_tbl_session = "session";
$phpAds_tbl_acls = "acls";
// The URL to your phpAds-installation
//$phpAds_url_prefix = "http://wpholub.worldonline.cz/redakce/banners";
//$phpAds_url_prefix = "/zadarmo/redakce/banners";
// Use INSERT DELAYED in logging functions?
$phpAds_insert_delayed = false;
// Your language file
$phpAds_language = "english";
// Enabled logging of adviews?
$phpAds_log_adviews = true;
// Enabled logging of adclicks?
$phpAds_log_adclicks = true;
// Admin's full name (used when sending stats via email)
$phpAds_admin_fullname = "it-media team";
// Admin's email address (used to set the FROM-address when sending email)
$phpAds_admin_email = "somebody@it-media.cz";
//Mail Headers for the reflection of the sender of the daily ad reports
$phpAds_admin_email_headers = "From: $phpAds_admin_email \n";
// The name of this application
$phpAds_name = "ZADARMO banners";
// Hosts to ignore (don't count adviews coming from them)
$phpAds_ignore_hosts = array(); // Example: array("slashdot.org", "microsoft.com");
// Reverse DNS lookup remotes hosts?
$phpAds_reverse_lookup = true;
// Disable magic_quotes_runtime - don't change
set_magic_quotes_runtime(0);
?>
function acl_check($request, $row) {
global $phpAds_tbl_acls;
global $phpAds_db;
$bannerID = $row['bannerID'];
if (($res = mysql_db_query($phpAds_db, "SELECT * FROM $phpAds_tbl_acls
WHERE bannerID = $bannerID ORDER by acl_order ")) == 0){
return(0);
}
while ($aclrow = mysql_fetch_array($res)) {
switch ($aclrow['acl_type']) {
case 'clientip':
$result = acl_check_clientip($request, $aclrow);
break;
case 'useragent':
$result = acl_check_useragent($request,
$aclrow);
break;
case 'weekday':
$result = acl_check_weekday($request,
$aclrow);
break;
default:
return(0);
}
if ($result != -1) {
return($result);
}
}
return(1);
}
function acl_check_weekday($request, $aclrow) {
$data = $aclrow['acl_data'];
$day = $request['weekday'];
if ($day == $data) {
switch ($aclrow['acl_ad']) {
case 'allow':
return(1);
case 'deny';
return(0);
default:
return(-1);
}
}
return(-1);
}
function acl_check_useragent($request, $aclrow) {
$data = $aclrow['acl_data'];
$agent = $request['user_agent'];
if (eregi($data, $agent)) {
switch ($aclrow['acl_ad']) {
case 'allow':
return(1);
case 'deny';
return(0);
default:
return(-1);
}
}
return(-1);
}
function acl_check_clientip($request, $aclrow) {
$data = $aclrow['acl_data'];
$host = $request['remote_host'];
list ($net, $mask) = explode('/', $data);
$net = explode('.', $net);
$pnet = pack('C4', $net[0], $net[1], $net[2], $net[3]);
$mask = explode('.', $mask);
$pmask = pack('C4', $mask[0], $mask[1], $mask[2], $mask[3]);
$host = explode('.', $host);
$phost = pack('C4', $host[0], $host[1], $host[2], $host[3]);
if (($phost & $pmask) == $pnet) {
switch ($aclrow['acl_ad']) {
case 'allow':
return(1);
case 'deny';
return(0);
default:
return(-1);
}
}
return(-1);
}
?>
/* $Id: lib.inc.php3,v 1.6 1999/12/15 09:18:15 tobias Exp $ */
// Display MySQL's last error message an die
function mysql_die()
{
global $strMySQLError;
echo "$strMySQLError: ";
echo mysql_error();
page_footer();
exit;
}
// Display a custom error message and die
function php_die($title="Error", $message="Unkown error")
{
?>
page_footer();
exit;
}
$link = mysql_pconnect($phpAds_hostname, $phpAds_mysqluser, $phpAds_mysqlpassword);
?>
// Get a banner
function get_banner($what, $clientID, $context=0)
{
global $phpAds_db, $REMOTE_HOST, $phpAds_tbl_banners, $REMOTE_ADDR, $HTTP_USER_AGENT;
static $displayed;
$where = "";
if($context == 0)
{
$context = array();
}
for($i=0; $i $value"; break;
case "==": $inclusive[] = "bannerID = $value"; break;
}
}
}
$where_exclusive = !empty($exclusive) ? implode(" AND ", $exclusive): "";
$where_inclusive = !empty($inclusive) ? implode(" OR ", $inclusive): "";
$where = sprintf("$where_inclusive %s $where_exclusive", (!empty($where_inclusive) && !empty($where_exclusive)) ? "AND": "");
$where = trim($where);
if(!empty($where))
{
$where .= " AND ";
}
$select = "SELECT
bannerID,
banner,
format,
width,
height,
alt,
bannertext,
url
FROM
$phpAds_tbl_banners
WHERE
$where
active = 'true' ";
if($clientID != 0)
{
$select .= " AND clientID = $clientID ";
}
if(is_string($what) && !ereg("[0-9]x[0-9]", $what))
{
switch($what)
{
// Get all HTML banners
case "html":
$select .= " AND format = 'html' ";
break;
//Not any of the special words (i.e. 'html'), So, must be a keyword
default:
$select .= " AND (";
$what_array = explode(",",$what);
// changed acording to the post from phpAds mailing list
//From: Wim Godden
//Date: Sat Jun 17, 2000 12:08pm
//Subject: [phpAds] Solution to 2 problems : keywords and limited banner selection
for($k=0; $k
*/
$select .= "keyword = 'global') ";
} //switch($what)
}
elseif(is_int($what))
{
$select .= " AND bannerID = $what ";
}
else
{
list($width, $height) = explode("x", $what);
// Get all banners with the specified width/height
$select .= " AND width = $width
AND height = $height ";
}
// print($select);
$res = @mysql_db_query($phpAds_db, $select);
if(!$res)
return(false);
$rows = array();
while ($tmprow = @mysql_fetch_array($res)) {
$rows[] = $tmprow;
}
$date = getdate(time());
$request = array('remote_host' => $REMOTE_ADDR,
'user_agent' => $HTTP_USER_AGENT,
'weekday' => $date['wday']);
count($rows) > 0 ? $cr = count($rows) : $cr = 1;
mt_srand((double)microtime()*1000000+ 1);
$randval = mt_rand() % $cr;
// this can be infinite loop that's why thereis check for $cnt
for($cnt=0; (!empty($displayed[$rows[$randval]["id"]])
or !acl_check($request, $rows[$randval]))
and $cnt<$cr*100; $cnt++)
{
$randval = mt_rand() % $cr;
}
$row = $rows[$randval];
$displayed[$row["id"]]=1;
return($row);
}
// Log an adview for the banner with $bannerID
function log_adview($bannerID)
{
global $phpAds_log_adviews, $phpAds_ignore_hosts, $phpAds_reverse_lookup, $phpAds_insert_delayed;
global $row, $phpAds_tbl_adviews;
global $REMOTE_HOST, $REMOTE_ADDR;
if(!$phpAds_log_adviews)
{
return(false);
}
if($phpAds_reverse_lookup)
{
$host = isset($REMOTE_HOST) ? $REMOTE_HOST : @gethostbyaddr($REMOTE_ADDR);
}
else
{
$host = $REMOTE_ADDR;
}
// Check if host is on list of hosts to ignore
$found = 0;
while(($found == 0) && (list($key, $ignore_host)=each($phpAds_ignore_hosts)))
{
if(eregi($ignore_host, $host)) // host found in ignore list
{
$found = 1;
}
}
if($found == 0)
{
$res = @mysql_db_query($GLOBALS["phpAds_db"], sprintf("
INSERT %s
INTO $phpAds_tbl_adviews
VALUES
(
'$bannerID',
null,
'$host'
)
", $phpAds_insert_delayed ? "DELAYED": ""));
}
}
// view a banner
function view($what, $clientID=0, $target = "", $withtext=0, $context=0)
{
global $phpAds_db, $REMOTE_HOST;
if(!is_int($clientID))
{
$target = $clientID;
$clientID = 0;
}
@mysql_pconnect($GLOBALS["phpAds_hostname"], $GLOBALS["phpAds_mysqluser"], $GLOBALS["phpAds_mysqlpassword"]);
$row = get_banner($what, $clientID, $context);
if(!empty($row["bannerID"]))
{
if(!empty($target))
{
$target = " target=\"$target\"";
}
if($row["format"] == "html")
{
if(!empty($row["url"]))
{
echo "";
}
echo $row["banner"];
if(!empty($row["url"]))
{
echo "";
}
}
else
{
echo " ";
if($withtext && !empty($row["bannertext"]))
{
echo " \n".$row["bannertext"]."";
}
}
}
log_adview($row["bannerID"]);
return($row["bannerID"]);
}
function view_t($what, $target = "")
{
view ($what, $target, 1);
}
?>
| |